101 Design Patterns & Tips for Developers
Creational Patterns
1.
Abstract Factory
The purpose of the Abstract Factory is to provide an interface for creating families of related objects, without specifying concrete classes (more...)
The purpose of the Abstract Factory is to provide an interface for creating families of related objects, without specifying concrete classes (more...)
2.
3.
Factory Method
The Factory Method defines an interface for creating objects, but lets subclasses decide which classes to instantiate (more...)
The Factory Method defines an interface for creating objects, but lets subclasses decide which classes to instantiate (more...)
4.
Object Pool
Object pools are used to manage the object caching and it can offer a significant performance boost (more...)
Object pools are used to manage the object caching and it can offer a significant performance boost (more...)
5.
Structural Patterns
7.
8.
9.
10.
11.
12.
13.
Private Class Data
The private class data design pattern seeks to reduce exposure of attributes by limiting their visibility (more...)
The private class data design pattern seeks to reduce exposure of attributes by limiting their visibility (more...)
Behavioral Patterns
15.
Chain of Responsibility
Chain of Responsibility chains the receiving objects together, and then passes any request messages from object to object until it reaches an object capable of handling the message (more...)
Chain of Responsibility chains the receiving objects together, and then passes any request messages from object to object until it reaches an object capable of handling the message (more...)
16.
17.
Interpreter
The Intepreter pattern defines a grammatical representation for a language and an interpreter to interpret the grammar (more...)
The Intepreter pattern defines a grammatical representation for a language and an interpreter to interpret the grammar (more...)
18.
19.
20.
21.
Null Object
The Null Object encapsulates the absence of an object by providing a substitutable alternative that offers suitable default "do nothing" behavior (more...)
The Null Object encapsulates the absence of an object by providing a substitutable alternative that offers suitable default "do nothing" behavior (more...)
22.
23.
24.
25.
Template Method
The Template Method defines a skeleton of an algorithm in an operation, and defers some steps to subclasses (more...)
The Template Method defines a skeleton of an algorithm in an operation, and defers some steps to subclasses (more...)
Composing Methods of Refactoring
27.
Extract Method
If you have a code fragment that can be grouped together, turn the fragment into a method whose name explains the purpose of the method (more...)
If you have a code fragment that can be grouped together, turn the fragment into a method whose name explains the purpose of the method (more...)
28.
Inline Method
If a method's body is just as clear as its name, put the method's body into the body of its callers and remove the method (more...)
If a method's body is just as clear as its name, put the method's body into the body of its callers and remove the method (more...)
29.
Inline Temp
If you have a temp that is assigned to once with a simple expression, and the temp is getting in the way of other refactorings, replace all references to that temp with the expression (more...)
If you have a temp that is assigned to once with a simple expression, and the temp is getting in the way of other refactorings, replace all references to that temp with the expression (more...)
30.
Introduce Explaining Variable
If you have a complicated expression, put the result of the expression, or parts of the expression, in a temporary variable with a name that explains the purpose (more...)
If you have a complicated expression, put the result of the expression, or parts of the expression, in a temporary variable with a name that explains the purpose (more...)
31.
Remove Assignments to Parameters
If the code assigns to a parameter, use a temporary variable instead (more...)
If the code assigns to a parameter, use a temporary variable instead (more...)
32.
Replace Method with Method Object
If uou have a long method that uses local variables in such a way that you cannot apply Extract Method, turn the method into its own object so that all the local variables become fields on that object (more...)
If uou have a long method that uses local variables in such a way that you cannot apply Extract Method, turn the method into its own object so that all the local variables become fields on that object (more...)
33.
Replace Temp with Query
If you are using a temporary variable to hold the result of an expression, extract the expression into a method. Replace all references to the temp with the expression. The new method can then be used in other methods (more...)
If you are using a temporary variable to hold the result of an expression, extract the expression into a method. Replace all references to the temp with the expression. The new method can then be used in other methods (more...)
34.
Split Temporary Variable
If you have a temporary variable assigned to more than once, but is not a loop variable nor a collecting temporary variable, make a separate temporary variable for each assignment (more...)
If you have a temporary variable assigned to more than once, but is not a loop variable nor a collecting temporary variable, make a separate temporary variable for each assignment (more...)
34.
Substitute Algorithm
If you want to replace an algorithm with one that is clearer, replace the body of the method with the new algorithm (more...)
If you want to replace an algorithm with one that is clearer, replace the body of the method with the new algorithm (more...)
Moving Features Between Objects
35.
Extract Class
If you have one class doing work that should be done by two, create a new class and move the relevant fields and methods from the old class into the new class (more...)
If you have one class doing work that should be done by two, create a new class and move the relevant fields and methods from the old class into the new class (more...)
36.
Hide Delegate
If a client is calling a delegate class of an object, create methods on the server to hide the delegate (more...)
If a client is calling a delegate class of an object, create methods on the server to hide the delegate (more...)
37.
Inline Class
If a class isn't doing very much, move all its features into another class and delete it (more...)
If a class isn't doing very much, move all its features into another class and delete it (more...)
38.
Introduce Foreign Method
If a server class you are using needs an additional method, but you can't modify the class, create a method in the client class with an instance of the server class as its first argument (more...)
If a server class you are using needs an additional method, but you can't modify the class, create a method in the client class with an instance of the server class as its first argument (more...)
39.
Introduce Local Extension
If a server class you are using needs several additional methods, but you can't modify the class, create a new class that contains these extra methods. Make this extension class a subclass or a wrapper of the original (more...)
If a server class you are using needs several additional methods, but you can't modify the class, create a new class that contains these extra methods. Make this extension class a subclass or a wrapper of the original (more...)
40.
Move Field
If a field is, or will be, used by another class more than the class on which it is defined, create a new field in the target class, and change all its users (more...)
If a field is, or will be, used by another class more than the class on which it is defined, create a new field in the target class, and change all its users (more...)
41.
Move Method
If a method is, or will be, using or used by more features of another class than the class on which it is defined, create a new method with a similar body in the class it uses most. Either turn the old method into a simple delegation, or remove it altogether (more...)
If a method is, or will be, using or used by more features of another class than the class on which it is defined, create a new method with a similar body in the class it uses most. Either turn the old method into a simple delegation, or remove it altogether (more...)
42.
Remove Middle Man
If a class is doing too much simple delegation, get the client to call the delegate directly (more...)
If a class is doing too much simple delegation, get the client to call the delegate directly (more...)
Organizing Data
43.
Change Bidirectional Association to Unidirectional
If you have a two-way association but one class no longer needs features from the other, drop the unneeded end of the association (more...)
If you have a two-way association but one class no longer needs features from the other, drop the unneeded end of the association (more...)
44.
Change Reference to Value
If you have a reference object that is small, immutable, and awkward to manage, turn it into a value object (more...)
If you have a reference object that is small, immutable, and awkward to manage, turn it into a value object (more...)
45.
Change Unidirectional Association to Bidirectional
If you have two classes that need to use each other's features, but there is only a one-way link, add back pointers, and change modifiers to update both sets (more...)
If you have two classes that need to use each other's features, but there is only a one-way link, add back pointers, and change modifiers to update both sets (more...)
46.
Change Value to Reference
If you have a class with many equal instances that you want to replace with a single object, turn the object into a reference object (more...)
If you have a class with many equal instances that you want to replace with a single object, turn the object into a reference object (more...)
47.
Duplicate Observed Data
If you have domain data available only in a GUI control, and domain methods need access, copy the data to a domain object. Set up an observer to synchronize the two pieces of data (more...)
If you have domain data available only in a GUI control, and domain methods need access, copy the data to a domain object. Set up an observer to synchronize the two pieces of data (more...)
48.
Encapsulate Collection
If a method returns a collection, make it return a read-only view and provide add/remove methods (more...)
If a method returns a collection, make it return a read-only view and provide add/remove methods (more...)
49.
50.
Replace Array with Object
If you have an array in which certain elements mean different things, replace the array with an object that has a field for each element (more...)
If you have an array in which certain elements mean different things, replace the array with an object that has a field for each element (more...)
51.
Replace Data Value with Object
If you have a data item that needs additional data or behavior, turn the data item into an object (more...)
If you have a data item that needs additional data or behavior, turn the data item into an object (more...)
52.
Replace Magic Number with Symbolic Constant
If you have a literal number with a particular meaning, create a constant, name it after the meaning, and replace the number with it (more...)
If you have a literal number with a particular meaning, create a constant, name it after the meaning, and replace the number with it (more...)
53.
Replace Record with Data Class
If you need to interface with a record structure in a traditional programming environment, make a dumb data object for the record (more...)
If you need to interface with a record structure in a traditional programming environment, make a dumb data object for the record (more...)
54.
Replace Subclass with Fields
If you have subclasses that vary only in methods that return constant data, change the methods to superclass fields and eliminate the subclasses (more...)
If you have subclasses that vary only in methods that return constant data, change the methods to superclass fields and eliminate the subclasses (more...)
55.
Replace Type Code with Class
If a class has a numeric type code that does not affect its behavior, replace the number with a new class (more...)
If a class has a numeric type code that does not affect its behavior, replace the number with a new class (more...)
56.
Replace Type Code with State/Strategy
If you have a type code that affects the behavior of a class, but you cannot use subclassing, replace the type code with a state object (more...)
If you have a type code that affects the behavior of a class, but you cannot use subclassing, replace the type code with a state object (more...)
57.
Replace Type Code with Subclasses
If you have an immutable type code that affects the behavior of a class, replace the type code with subclasses (more...)
If you have an immutable type code that affects the behavior of a class, replace the type code with subclasses (more...)
58.
Introduce Explaining Variable
If you have a complicated expression, put the result of the expression, or parts of the expression, in a temporary variable with a name that explains the purpose (more...)
If you have a complicated expression, put the result of the expression, or parts of the expression, in a temporary variable with a name that explains the purpose (more...)
59.
Remove Assignments to Parameters
If the code assigns to a parameter, use a temporary variable instead (more...)
If the code assigns to a parameter, use a temporary variable instead (more...)
60.
Self Encapsulate Field
If you are accessing a field directly, but the coupling to the field is becoming awkward, create getting and setting methods for the field and use only those to access the field (more...)
If you are accessing a field directly, but the coupling to the field is becoming awkward, create getting and setting methods for the field and use only those to access the field (more...)
Simplifying Conditional Expressions
61.
Consolidate Conditional Expression
If you have a sequence of conditional tests with the same result, combine them into a single conditional expression and extract it (more...)
If you have a sequence of conditional tests with the same result, combine them into a single conditional expression and extract it (more...)
62.
Consolidate Duplicate Conditional Fragments
If the same fragment of code is in all branches of a conditional expression, move it outside of the expression (more...)
If the same fragment of code is in all branches of a conditional expression, move it outside of the expression (more...)
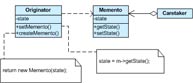
63.
Decompose Conditional
If you have a complicated conditional (if-then-else) statement, extract methods from the condition, then part, and else parts (more...)
If you have a complicated conditional (if-then-else) statement, extract methods from the condition, then part, and else parts (more...)
64.
Introduce Assertion
If a section of code assumes something about the state of the program, make the assumption explicit with an assertion (more...)
If a section of code assumes something about the state of the program, make the assumption explicit with an assertion (more...)
65.
Introduce Null Object
If you have repeated checks for a null value, replace the null value with a null object (more...)
If you have repeated checks for a null value, replace the null value with a null object (more...)
66.
Remove Control Flag
If you have a variable that is acting as a control flag for a series of boolean expressions, use a break or return instead (more...)
If you have a variable that is acting as a control flag for a series of boolean expressions, use a break or return instead (more...)
67.
Replace Conditional with Polymorphism
If you have a conditional that chooses different behavior depending on the type of an object, move each leg of the conditional to an overriding method in a subclass. Make the original method abstract (more...)
If you have a conditional that chooses different behavior depending on the type of an object, move each leg of the conditional to an overriding method in a subclass. Make the original method abstract (more...)
68.
Replace Nested Conditional with Guard Clauses
If a method has conditional behavior that does not make clear the normal path of execution, use guard clauses for all the special cases (more...)
If a method has conditional behavior that does not make clear the normal path of execution, use guard clauses for all the special cases (more...)
Making Method Calls Simpler
69.
Add Parameter
If a method needs more information from its caller, add a parameter for an object that can pass on this information (more...)
If a method needs more information from its caller, add a parameter for an object that can pass on this information (more...)
70.
Encapsulate Downcast
If a method returns an object that needs to be downcasted by its callers, move the downcast to within the method (more...)
If a method returns an object that needs to be downcasted by its callers, move the downcast to within the method (more...)
71.
72.
Introduce Parameter Object
If you have a group of parameters that naturally go together, replace them with an object (more...)
If you have a group of parameters that naturally go together, replace them with an object (more...)
73.
Hide Delegate
If a client is calling a delegate class of an object, create methods on the server to hide the delegate (more...)
If a client is calling a delegate class of an object, create methods on the server to hide the delegate (more...)
74.
Parameterize Method
If several methods do similar things but with different values contained in the method body, create one method that uses a parameter for the different values (more...)
If several methods do similar things but with different values contained in the method body, create one method that uses a parameter for the different values (more...)
75.
Preserve Whole Object
If you are getting several values from an object and passing these values as parameters in a method call, send the whole object instead (more...)
If you are getting several values from an object and passing these values as parameters in a method call, send the whole object instead (more...)
76.
77.
Remove Setting Method
A field should be set at creation time and never altered. Remove any setting method for that field (more...)
A field should be set at creation time and never altered. Remove any setting method for that field (more...)
78.
Rename Method
If the name of a method does not reveal its purpose, change the name of the method (more...)
If the name of a method does not reveal its purpose, change the name of the method (more...)
79.
Replace Constructor with Factory Method
If you want to do more than simple construction when you create an object, replace the constructor with a factory method (more...)
If you want to do more than simple construction when you create an object, replace the constructor with a factory method (more...)
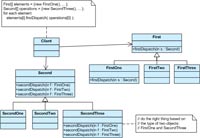
80.
Replace Error Code with Exception
If a method returns a special code to indicate an error, throw an exception instead (more...)
If a method returns a special code to indicate an error, throw an exception instead (more...)
81.
Replace Exception with Test
If you are throwing a checked exception on a condition the caller could have checked first, change the caller to make the test first (more...)
If you are throwing a checked exception on a condition the caller could have checked first, change the caller to make the test first (more...)
82.
Replace Parameter with Explicit Methods
If you have a method that runs different code depending on the values of an enumerated parameter, create a separate method for each value of the parameter (more...)
If you have a method that runs different code depending on the values of an enumerated parameter, create a separate method for each value of the parameter (more...)
83.
Replace Parameter with Method
If an object invokes a method, then passes the result as a parameter for a method. The receiver can also invoke this method, remove the parameter and let the receiver invoke the method (more...)
If an object invokes a method, then passes the result as a parameter for a method. The receiver can also invoke this method, remove the parameter and let the receiver invoke the method (more...)
84.
Separate Query from Modifier
If you have a method that returns a value but also changes the state of an object, create two methods, one for the query and one for the modification (more...)
If you have a method that returns a value but also changes the state of an object, create two methods, one for the query and one for the modification (more...)
Dealing with Generalization
85.
Collapse Hierarchy
If a superclass and subclass are not very different, merge them together (more...)
If a superclass and subclass are not very different, merge them together (more...)
86.
Extract Interface
If several clients use the same subset of a class's interface, or two classes have part of their interfaces in common, extract the subset into an interface (more...)
If several clients use the same subset of a class's interface, or two classes have part of their interfaces in common, extract the subset into an interface (more...)
87.
Extract Subclass
If a class has features that are used only in some instances, create a subclass for that subset of features (more...)
If a class has features that are used only in some instances, create a subclass for that subset of features (more...)
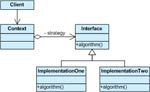
88.
Replace Array with Object
If you have an array in which certain elements mean different things, replace the array with an object that has a field for each element (more...)
If you have an array in which certain elements mean different things, replace the array with an object that has a field for each element (more...)
89.
Replace Data Value with Object
If you have a data item that needs additional data or behavior, turn the data item into an object (more...)
If you have a data item that needs additional data or behavior, turn the data item into an object (more...)
90.
Extract Superclass
If you have two classes with similar features, create a superclass and move the common features to the superclass (more...)
If you have two classes with similar features, create a superclass and move the common features to the superclass (more...)
91.
Form Template Method
If you have two methods in subclasses that perform similar steps in the same order, yet the steps are different, get the steps into methods with the same signature, so that the original methods become the same. Then you can pull them up (more...)
If you have two methods in subclasses that perform similar steps in the same order, yet the steps are different, get the steps into methods with the same signature, so that the original methods become the same. Then you can pull them up (more...)
92.
Pull Up Constructor Body
If you have constructors on subclasses with mostly identical bodies, create a superclass constructor; call this from the subclass methods (more...)
If you have constructors on subclasses with mostly identical bodies, create a superclass constructor; call this from the subclass methods (more...)
93.
94.
Pull Up Method
If you have methods with identical results on subclasses, move them to the superclass (more...)
If you have methods with identical results on subclasses, move them to the superclass (more...)
95.
Push Down Field
If a field is used only by some subclasses, move the field to those subclasses (more...)
If a field is used only by some subclasses, move the field to those subclasses (more...)
96.
Push Down Method
If behavior on a superclass is relevant only for some of its subclasses, move it to those subclasses (more...)
If behavior on a superclass is relevant only for some of its subclasses, move it to those subclasses (more...)
97.
Replace Delegation with Inheritance
If you're using delegation and are often writing many simple delegations for the entire interface, make the delegating class a subclass of the delegate (more...)
If you're using delegation and are often writing many simple delegations for the entire interface, make the delegating class a subclass of the delegate (more...)
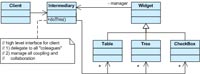
98.
Replace Inheritance with Delegation
If a subclass uses only part of a superclasses interface or does not want to inherit data, create a field for the superclass, adjust methods to delegate to the superclass, and remove the subclassing (more...)
If a subclass uses only part of a superclasses interface or does not want to inherit data, create a field for the superclass, adjust methods to delegate to the superclass, and remove the subclassing (more...)
Big Refactorings
99.
Convert Procedural Design to Objects
If you have code written in a procedural style, turn the data records into objects, break up the behavior, and move the behavior to the objects (more...)
If you have code written in a procedural style, turn the data records into objects, break up the behavior, and move the behavior to the objects (more...)
100.
Extract Hierarchy
If you have a class that is doing too much work, at least in part through many conditional statements, create a hierarchy of classes in which each subclass represents a special case (more...)
If you have a class that is doing too much work, at least in part through many conditional statements, create a hierarchy of classes in which each subclass represents a special case (more...)
101.
Tease Apart Inheritance
If you have an inheritance hierarchy that is doing two jobs at once, create two hierarchies and use delegation to invoke one from the other (more...)
If you have an inheritance hierarchy that is doing two jobs at once, create two hierarchies and use delegation to invoke one from the other (more...)